C++?????????????10??C++11????
???????????? ???????[ 2014/11/26 11:37:42 ] ????????C++ ????? ???????
??????????begin()??end()????
??????????????????????????????????????????begin()??end()??????????????????????????????????????????????ù?????????д????????????????????????????е?STL????????????????????????????????κε?????????????????C?????????????
??????????????????????vector????????????????????????????????std::vector???黻??C??????飬????????????????????
????1 int arr[] = {1??2??3};
????2 std::for_each(&arr[0]?? &arr[0]+sizeof(arr)/sizeof(arr[0])?? [](int n) {std::cout << n << std::endl;});
????3
????4 auto is_odd = [](int n) {return n%2==1;};
????5 auto begin = &arr[0];
????6 auto end = &arr[0]+sizeof(arr)/sizeof(arr[0]);
????7 auto pos = std::find_if(begin?? end?? is_odd);
????8 if(pos != end)
????9 std::cout << *pos << std::endl;
????????????????begin()??end()????????????????
1 int arr[] = {1??2??3};
2 std::for_each(std::begin(arr)?? std::end(arr)?? [](int n) {std::cout << n << std::endl;});
3
4 auto is_odd = [](int n) {return n%2==1;};
5 auto pos = std::find_if(std::begin(arr)?? std::end(arr)?? is_odd);
6 if(pos != std::end(arr))
7 std::cout << *pos << std::endl;
??δ????std::vector?汾?????????????????????????????begin()??end()??????????д????????????
1 template <typename Iterator>
2 void bar(Iterator begin?? Iterator end)
3 {
4 std::for_each(begin?? end?? [](int n) {std::cout << n << std::endl;});
5
6 auto is_odd = [](int n) {return n%2==1;};
7 auto pos = std::find_if(begin?? end?? is_odd);
8 if(pos != end)
9 std::cout << *pos << std::endl;
10 }
11
12 template <typename C>
13 void foo(C c)
14 {
15 bar(std::begin(c)?? std::end(c));
16 }
17
18 template <typename T?? size_t N>
19 void foo(T(&arr)[N])
20 {
21 bar(std::begin(arr)?? std::end(arr));
22 }
23
24 int arr[] = {1??2??3};
25 foo(arr);
26
27 std::vector<int> v;
28 v.push_back(1);
29 v.push_back(2);
30 v.push_back(3);
31 foo(v);
????????????????????
???????????????????????ζ????顣?????????棬????????????????????????????????????????????
????1 template <typename T?? size_t Size>
????2 class Vector
????3 {
????4 static_assert(Size < 3?? "Size is too small");
????5 T _points[Size];
????6 };
????7
????8 int main()
????9 {
????10 Vector<int?? 16> a1;
????11 vector<double?? 2> a2;
????12 return 0;
????13 }
????error C2338: Size is too small
????see reference to class template instantiation 'Vector<T??Size>' being compiled
????with
????[
????T=double??
????Size=2
????]
???????????????????????????y??????????ν????????????????????????????????????????λ??????<type_traits>?С?????????ж?????ü???????????????????????????????????????????????????????????????????????????????????????????????????μ??????
????????????????У?add??????????趨????????????
????1 template <typename T1?? typename T2>
????2 auto add(T1 t1?? T2 t2) -> decltype(t1 + t2)
????3 {
????4 return t1 + t2;
????5 }
??????????????????д???????????????
????1 std::cout << add(1?? 3.14) << std::endl;
????2 std::cout << add("one"?? 2) << std::endl;
????????????????????4.14??"e"??????????????????????????????д??????±????????
1 template <typename T1?? typename T2>
2 auto add(T1 t1?? T2 t2) -> decltype(t1 + t2)
3 {
4 static_assert(std::is_integral<T1>::value?? "Type T1 must be integral");
5 static_assert(std::is_integral<T2>::value?? "Type T2 must be integral");
6
7 return t1 + t2;
8 }
error C2338: Type T2 must be integral
see reference to function template instantiation 'T2 add<int??double>(T1??T2)' being compiled
with
[
T2=double??
T1=int
]
error C2338: Type T1 must be integral
see reference to function template instantiation 'T1 add<const char*??int>(T1??T2)' being compiled
with
[
T1=const char *??
T2=int
]
???????????
??????????????????C++11????????????????????????д?ü??????????????????????????????????????????????????????????????????????????????????????
????C++11??????????????????&&????????????????????????????????????????????о?????????????????о??????????????????????????????????????????????????????????const T&?????????????
????C++??????????????Щ????????????????????????е???????????????????????????????????????????????????????????????????????????????????????????а?λ????????????????????λ??????????????????????Щ?????????????????????????????????????????????????????Щ???????????OK????????????????????????????????????????????????????????????????????????????????????д???????????????????????????????????
???????????????г?????????????????????????????????????????????????????????????????????????????????ζ??????????????濽??????????в??????????????б?????
????????????????????????????????????????????????????????????T&&???????????????????????????????????????????????????“???”??????????????????????磬?????????????vector????queue??????????????????????????????????????????????????????????????????????????????飬????????п????????????????????????????????????檔?????????????????????????????????????????????????楨????????????????????????????
??????????????????????????????????????????????????????????????T??????????????????std::unique_ptr?У???????????洢???鳤????????
1 template <typename T>
2 class Buffer
3 {
4 std::string _name;
5 size_t _size;
6 std::unique_ptr<T[]> _buffer;
7
8 public:
9 // default constructor
10 Buffer():
11 _size(16)??
12 _buffer(new T[16])
13 {}
14
15 // constructor
16 Buffer(const std::string& name?? size_t size):
17 _name(name)??
18 _size(size)??
19 _buffer(new T[size])
20 {}
21
22 // copy constructor
23 Buffer(const Buffer& copy):
24 _name(copy._name)??
25 _size(copy._size)??
26 _buffer(new T[copy._size])
27 {
28 T* source = copy._buffer.get();
29 T* dest = _buffer.get();
30 std::copy(source?? source + copy._size?? dest);
31 }
32
33 // copy assignment operator
34 Buffer& operator=(const Buffer& copy)
35 {
36 if(this != ©)
37 {
38 _name = copy._name;
39
40 if(_size != copy)
41 {
42 _buffer = nullptr;
43 _size = copy._size;
44 _buffer = _size > 0 ? new T[_size] : nullptr;
45 }
46
47 T* source = copy._buffer.get();
48 T* dest = _buffer.get();
49 std::copy(source?? source + copy._size?? dest);
50 }
51
52 return *this;
53 }
54
55 // move constructor
56 Buffer(Buffer&& temp):
57 _name(std::move(temp._name))??
58 _size(temp._size)??
59 _buffer(std::move(temp._buffer))
60 {
61 temp._buffer = nullptr;
62 temp._size = 0;
63 }
64
65 // move assignment operator
66 Buffer& operator=(Buffer&& temp)
67 {
68 assert(this != &temp); // assert if this is not a temporary
69
70 _buffer = nullptr;
71 _size = temp.size;
72 _buffer = std::move(temp._buffer);
73
74 _name = std::move(temp._name);
75
76 temp._buffer = nullptr;
77 temp._size = 0;
78
79 return *this;
80 }
81 };
82
83 template <typename T>
84 Buffer<T> getBuffer(const std::string& name)
85 {
86 Buffer<T> b(name?? 128);
87 return b;
88 }
89
90 int main()
91 {
92 Buffer<int> b1;
93 Buffer<int> b2("buf2"?? 64);
94 Buffer<int> b3 = b2;
95 Buffer<int> b4 = getBuffer<int>("buf4");
96 b1 = getBuffer<int>("buf5");
97 return 0;
98 }
??????
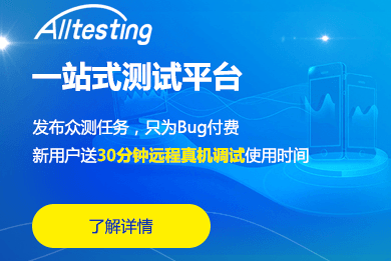
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11